Read the node properties¶
Problem Description¶
In this example we will read the nodes data from the database.
Following values are extracted:
NR
(node number)INR
(internal node number)KFIX
(degree of freedoms, bitwise encoded)NCOD
(additional bit code)X
(global X coordinate)Y
(global Y coordinate)Z
(global Z coordinate)
The nodes description can be found in CDBASE.CHM as shown in figure below:
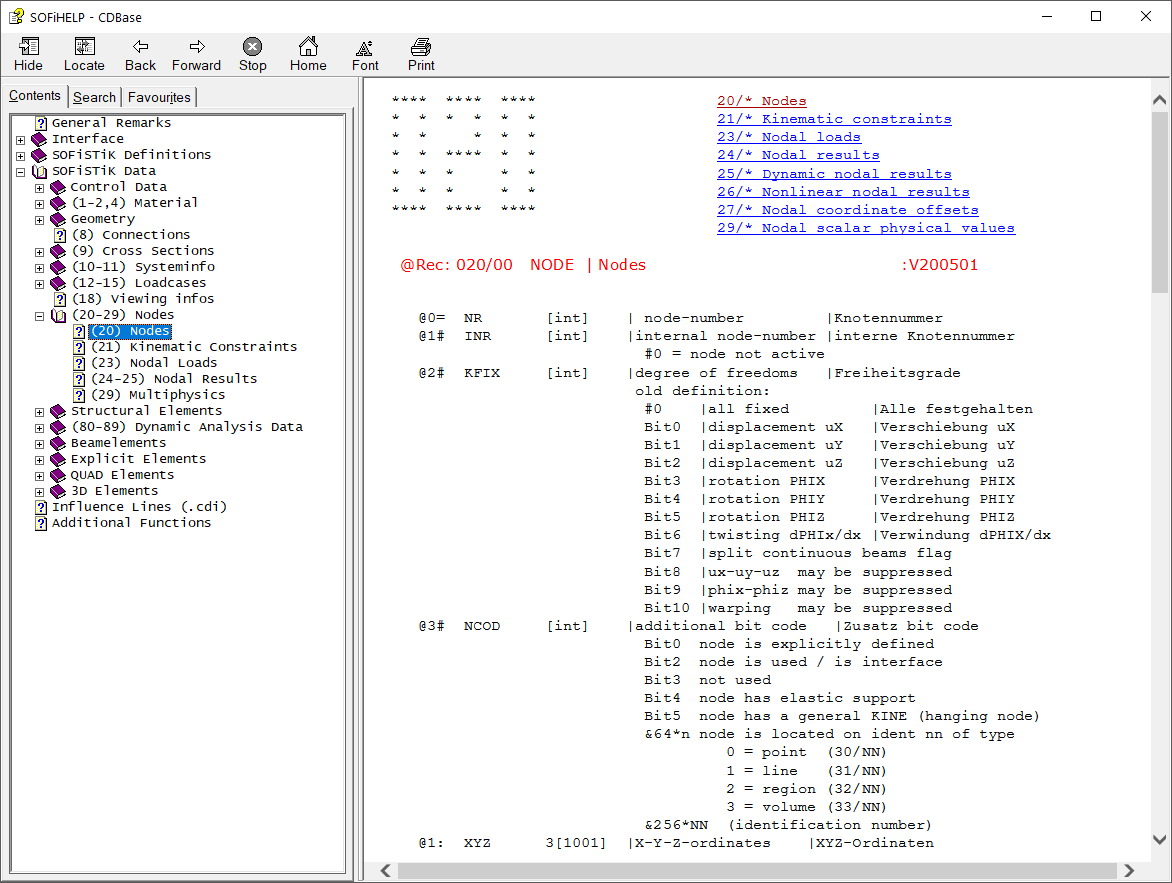
The [INT]
means that the data-type is integer. The data-type can easily be checked in the
data-type list given in the example.
Probem Solution¶
Lets read the nodes from the CDB. Create a new module and name it for example nodes and copy the code:
'Read the nodes from CDB
Public Sub readNodes()
Dim Filename As String
Dim Index, datalen As Long
Dim row, pos As Long
Dim arr_20_0() As Variant
ReDim arr_20_0(6, 0)
Dim Destination1 As Range
'Lets clear the sheet first
tab1.Cells.Clear
'The path of the file
Filename = tab1.TextBox1.Text
'To connect to the CDB we will use
Index = sof_cdb_init(Filename, 99)
'Get the data from CDB
Dim data As CNODE
datalen = Len(data)
Do While sof_cdb_get(Index, 20, 0, data, datalen, pos) = 0
row = row + 1
pos = pos + 1
'Redim the array for the output
ReDim Preserve arr_20_0(6, row)
'Copy the values to array
arr_20_0(0, row) = data.m_NR
arr_20_0(1, row) = data.m_INR
arr_20_0(2, row) = data.m_KFIX
arr_20_0(3, row) = data.m_NCOD
arr_20_0(4, row) = data.m_XYZ(1)
arr_20_0(5, row) = data.m_XYZ(2)
arr_20_0(6, row) = data.m_XYZ(3)
'Get the length of the data. This is very important!!!
datalen = Len(data)
Loop
'Set the names of header row and columns
arr_20_0(0, 0) = "NR"
arr_20_0(1, 0) = "INR"
arr_20_0(2, 0) = "KFIX"
arr_20_0(3, 0) = "NCOD"
arr_20_0(4, 0) = "X"
arr_20_0(5, 0) = "Y"
arr_20_0(6, 0) = "Z"
'Output the values to range
Set Destination1 = tab1.Range("A4")
Destination1.Resize(UBound(arr_20_0, 2) + 1, UBound(arr_20_0, 1) + 1).Value = Application.Transpose(arr_20_0)
'Center the output -> this is just for better looking
tab1.Cells.HorizontalAlignment = xlCenter
'Close the CDB
Call sof_cdb_close(0)
End Sub
Just run the Excel Workbook and the readNodes()
Sub.
Tip
To avoid a performance issue in Excel, do NOT write every value to every cell ( connect, write, connect, write… ). In our example we are storing all data into an dynamic array. At end, the data is written in one step to a range. This approach is much faster.
CNODE
is the data-type for the nodes in the CDB.sof_data
module.The example can be found by following:
C:\<sofistik_installation>\2020\SOFiSTiK 2020\interfaces\examples\vba\read_nodes